In this tutorial we going to learn how to delete a node at given position from linkedlist . if you are getting how it's working then please see once our previous post of this topic..
Saturday, 10 February 2018
Delete a Linked List node at a given position
Delete a Linked List node,Delete a Linked List node at a given position,Delete a Linked List node at a given position in java,delete a node in data structure, how to delete a node,how to delete a node in java,
Delete a Linked List node:
In this tutorial we going to learn how to delete a node at given position from linkedlist . if you are getting how it's working then please see once our previous post of this topic..
if you are getting how it's working then please see once our previous post of this topic..
In this tutorial we going to learn how to delete a node at given position from linkedlist . if you are getting how it's working then please see once our previous post of this topic..
package com.dashzin.deletion;
public class DeleteNode {
Node head;
static class Node{
int data;
Node next;
Node(int data){
this.data=data;
next=null;
}
}
/* This method will prints data of linked list starting
from head */
public void printList(){
while(head!=null){
System.out.print(head.data+" ");
head=head.next;
}
}
/* Inserts a new Node at front of the list. */
public void insert(int new_data){
Node new_node=new Node(new_data);
new_node.next=head;
head=new_node;
}
/* Given a key, deletes the first occurrence of key in
linked list */
void deleteNode(int position){
// checking list is empty...
if(head==null){
return;
}
// store the head
Node temp=head;
// If head needs to be removed
if(position==0){
head=temp.next;//change head...
return;
}
// Find previous node of the node to be deleted
for (int i=0; temp!=null && i<position-1; i++)
temp = temp.next;
// If position is more than number of nodes
if (temp == null || temp.next == null)
return;
// Node temp->next is the node to be deleted
// Store pointer to the next of node to be deleted
Node next = temp.next.next;
temp.next = next; // Unlink the deleted node from list
}
public static void main(String args[]){
DeleteNode lkl=new DeleteNode();
lkl.insert(5);
lkl.insert(10);
lkl.insert(20);
lkl.insert(100);
lkl.insert(30);
lkl.printList();
lkl.deleteNode(2); // Delete node at position 2
System.out.println(".................");
lkl.printList();
}
}
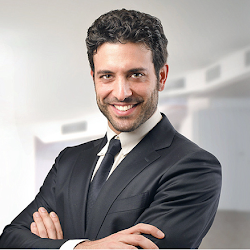
DashZin
Author & Editor
Has laoreet percipitur ad. Vide interesset in mei, no his legimus verterem. Et nostrum imperdiet appellantur usu, mnesarchum referrentur id vim.
February 10, 2018
Data Structures, LinkedList
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment