ArrayList
|
LinkList
|
Every Method Present Inside
ArrayListis Non – Synchronized.
|
Relatively Performance is High because
Threads are Not required to Wait
|
At a Time Multiple Threads are allow
to Operate on ArrayList Simultaneously and Hence ArrayList Object is Not
Thread Safe
|
At a Time Only One Thread is allow to
Operate on Vector Object and Hence Vector Object is Always Thread Safe.
|
Relatively Performance is High because
Threads are Not required to Wait
|
Relatively Performance is Low because
Threads are required to Wait.
|
Thursday, 1 February 2018
List in Collection
Java Collections, Collection in java, Java Collections Framework Tutorials, ArrayList , LinkedList,Vector , HashSet ,LinkedHashSet , TreeSet , HashMap ,TreeMap , LinkedHashMap , Hashtable , Iterator and ListIterator , Comparable and Comparator ,Java Collections Interview Questions
It is the Child Interface of Collection. If we want to
Represent a Group of Individual Objects where Duplicates are allowed and
Insertion Order Preserved. Then we should go for List. We can Preserve
Insertion Order and we can Differentiate Duplicate Object by using Index. Hence
Index will Play Very Important Role in List.
Methods:
List Interface Defines the following Specific Methods.
1) void add(int
index, Object o)
2)
booleanaddAll(int index, Collection c)
3) Object get(int
index)
4) Object
remove(int index)
5) Object set(int
index, Object new): To Replace the Element Present at specified Index with provided Object and Returns Old Object.
6)
intindexOf(Object o):Returns Index of 1st Occurrence of 'o'
7)
intlastIndexOf(Object o)
8)
ListIteratorlistIterator();
ArrayList: -
The Underlying Data Structure for ArrayList is Resizable
Array ORGrowable Array. Duplicate Objects are allowed. Insertion Order is
Preserved. Heterogeneous Objects are
allowed (Except TreeSet and TreeMap Everywhere Heterogeneous Objects are
allowed). null Insertion is Possible.
Constructors:
1)
ArrayList
l = new ArrayList();
Creates
an Empty ArrayList Object with Default Initial Capacity 10. If ArrayList Reaches its Max Capacity then a
New ArrayList Object will be Created with
2) ArrayList l = new ArrayList(intinitialCapacity);
Creates an Empty ArrayList Object
with specified Initial Capacity.
3) ArrayList l = new ArrayList(Collection c);
Example : -
importjava.util.ArrayList;
classArrayListDemo
{
public static void
main(String[] args)
{
ArrayList l = new ArrayList();
l.add("A");
l.add(10);
l.add("A");
l.add(null);
System.out.println(l);
//[A, 10, A, null]
l.remove(2); System.out.println(l); //[A, 10, null]
l.add(2,"M");
l.add("N");
System.out.println(l);
//[A, 10, M, null, N]
}
}
·
Usually we can Use Collections to Hold and
Transfer Data (Objects) form One Location to Another Location.
·
To Provide Support for this Requirement Every
Collection Class Implements Serializable and Cloneable Interfaces.
·
ArrayList and Vector Classes Implements
RandomAccess Interface. So that we can Access any Random Element with the Same
Speed.
·
RandomAccess Interface Present in
java.utilPackage and it doesn't contain any Methods. Hence it is a Marker
Interface.
·
Hence ArrayList is Best Suitable if Our Frequent
Operation is Retrieval Operation.
ArrayList l1 = new ArrayList();
LinkedList l2 = new LinkedList();
System.out.println(l1
instanceofSerializable); //true
System.out.println(l2
instanceofCloneable); //true
System.out.println(l1
instanceofRandomAccess); //true
System.out.println(l2
instanceofRandomAccess); //false
Differences between ArrayList and
Vector:
Que: How to get Synchronized Version of ArrayList Object?
By
Default ArrayList Object is Non- Synchronized but we can get Synchronized
Version ArrayList Object by using the following Method of Collections Class.
public static List synchronizedList(List l)
example:-
ArrayListal = new ArrayList ();
List l = Collections.synchronizedList(al);
Where :-
l=> is Synchronized Version
al=> is Non - Synchronized Version
Similarly
we can get Synchronized Version of Set and Map Objects by using the following
Methods of Collection Class.
public static Set synchronizedSet(Set s)
public static Map
synchronizedMap(Map m)
·
ArrayList is the Best Choice if we want to
Perform Retrieval Operation Frequently.
·
But ArrayList is Worst Choice if Our Frequent
Operation is Insertion OR Deletion in the Middle. Because it required Several
Shift Operations Internally.
LinkedList:
o
The Underlying Data Structure is Double
LinkedList.
o
Insertion Order is Preserved.
o
Duplicate Objects are allowed.
o
Heterogeneous Objects are allowed.
o
null Insertion is Possible.
o
Implements Serializable and Cloneable Interfaces
but Not RandomAccessInterface.
o
Best Choice if Our Frequent Operation is
Insertion OR Deletion in the Middle.
o
Worst Choice if Our Frequent Operation is
Retrieval.
Constructors:
1) LinkedList l = new LinkedList(); Creates an Empty
LinkedList Object.
2) LinkedList l = new LinkedList(Collection c); Creates an
Equivalent LinkedList Object for the given Collection.
Methods: Usually we can Use LinkedList to Implement Stacks and
Queues. To Provide Support for this Requirement LinkedList Class Defines the
following 6 Specific Methods.
1) void addFirst(Object o)
2) void addLast(Object o)
3) Object getFirst()
4) Object getLast()
5) Object removeFirst()
6) Object removeLast()
Example :-
importjava.util.LinkedList;
classLinkedListDemo
{
public static void main(String[]
args)
{
LinkedList l = new
LinkedList();
l.add("DashZin");
l.add(30);
l.add(null);
l.add("DashZin");
l.set(0, "Software");
l.add(0,"Info");
l.removeLast();
l.addFirst("CCC");
System.out.println(l); //[CCC, Info,
Software, 30, null]
}
}
Vector:
·
The Underlying Data Structure is Resizable Array
ORGrowable Array.
·
Insertion Order is Preserved.
·
Duplicate Objects are allowed.
·
Heterogeneous Objects are allowed.
·
null Insertion is Possible.
·
Implements Serializable, Cloneable and RandomAccess
interfaces.
·
Every Method Present Inside Vector is
Synchronized and Hence Vector Object is Thread Safe.
·
Vector is the Best Choice if Our Frequent
Operation is Retrieval.
·
Worst Choice if Our Frequent Operation is
Insertion OR Deletion in the Middle.
Constructors:
1) Vector v = new Vector();
Creates an Empty Vector Object with Default Initial Capacity 10.
Once Vector Reaches its Max Capacity then a New Vector Object will be
Created with
New Capacity = Current Capacity * 2
2) Vector v = new
Vector(intinitialCapacity);
3) Vector v = new
Vector(intinitialCapacity, intincrementalCapacity);
4) Vector v = new
Vector(Collection c);
Example:-
Import java.util.Vector;
classVectorDemo
{
public static void main(String[]
args)
{
Vector v = new Vector();
System.out.println(v.capacity());
//10
for(int i = 1; i<=10; i++) {
v.addElement(i);
}
System.out.println(v.capacity());
//10
v.addElement("A");
System.out.println(v.capacity());
//20
System.out.println(v); //[1, 2, 3,
4, 5, 6, 7, 8, 9, 10, A]
}
}
Stack:
It is the Child Class of Vector.
It is a Specially Designed Class for Last In First Out (LIFO) Order.
Constructor:Stack s = new Stack();
Methods:
1)
Object push(Object o); => To Insert an Object into the
Stack.
2)
Object pop(); => To Remove and Return Top of the Stack.
3)
Object peek(); => Ro Return Top of the Stack without
Removal.
4)
boolean empty(); => Returns true if Stack is Empty
5)
int search(Object o); =>Returns Offset if the Element is
Available Otherwise Returns -1.
importjava.util.Stack;
classStackDemo
{
public
static void main(String[] args)
{
Stack s =
new Stack();
s.push("A");
s.push("B");
s.push("C");
System.out.println(s);
//[A, B, C]
System.out.println(s.search("A"));
//3
System.out.println(s.search("Z"));
//-1
}
}
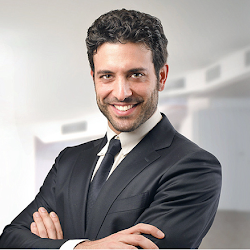
DashZin
Author & Editor
Has laoreet percipitur ad. Vide interesset in mei, no his legimus verterem. Et nostrum imperdiet appellantur usu, mnesarchum referrentur id vim.
February 01, 2018
java, Java Collections, Java technology
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment